ADVANCED AUTOMATED COPYING FROM EXTERNAL FLASH DRIVES TO LOCAL DISK IN WINDOWS ENVIRONMENT
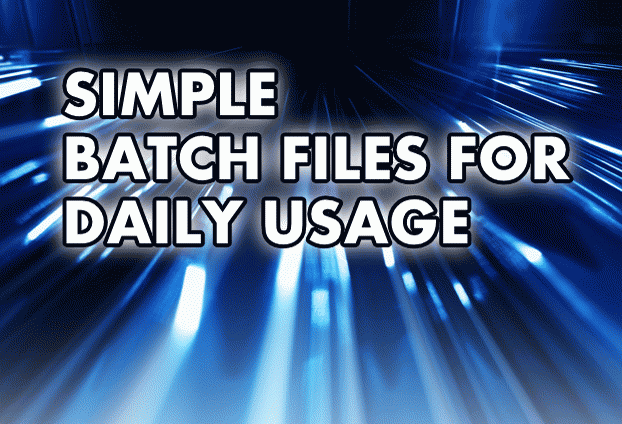
Today we are going to make a deep dive into advanced file management and automation.
There are several scenarios for copying files from external storage that are described here.
► COPY SPECIFIC FILE TYPES FROM MULTIPLE FOLDERS ON ANY EXTERNAL DRIVE TO THE LOCAL HARD DRIVE
This script will copy ALL JPG FILES from folders TEST1 and TEST2,
which are located on any flash-drive to folder 1234 located on local drive C.
@echo off
setlocal enabledelayedexpansion
:: Local destination folder
set "destFolder=C:\1234"
:: Create the destination folder if it doesn't exist
if not exist "%destFolder%" (
mkdir "%destFolder%"
)
:: Loop through all drives to find removable drives
for %%D in (C D E F G H I J K L M N O P Q R S T U V W X Y Z) do (
:: Check if the drive is removable
wmic logicaldisk where "DeviceID='%%D:' and DriveType=2" get DeviceID 2>nul | find "%%D:" >nul
if !errorlevel! equ 0 (
:: Check if the "test1" folder exists on the drive
if exist "%%D:\test1\" (
echo Copying files from %%D:\test1\
xcopy "%%D:\test1\*.jpg" "%destFolder%" /s /i /y
)
:: Check if the "test2" folder exists on the drive
if exist "%%D:\test2\" (
echo Copying files from %%D:\test2\
xcopy "%%D:\test2\*.jpg" "%destFolder%" /s /i /y
)
)
)
echo File copying complete.
pause
► COPY SPECIFIC FILE TYPES FROM A SPECIFIC FOLDER ON A SPECIFIC FLASH DRIVE TO THE LOCAL HARD DRIVE
Upcoming script could be helpful if you are for some reason worried about origin of files and
from what external drive they came from.
To make this happen you need to know precise id number of your external drive. This could be done like this:
vol X:
where X is the letter of your drive.
System will report something like:
E11C-38E6
In provided script JPG, DNG, ORF files will be copied from 4UPLOAD folder
located on external drive to 1234 folder located on local drive C,
but ONLY if the drive has serial number E11C-38E6.
@echo off
setlocal enabledelayedexpansion
:: Serial number of the flash drive
set "targetSerial=E11C-38E6"
:: Local destination folder
set "destFolder=C:\1234"
:: Create the destination folder if it doesn't exist
if not exist "%destFolder%" (
mkdir "%destFolder%"
)
:: Loop through all drives and check serial number using 'vol' command
for %%D in (C D E F G H I J K L M N O P Q R S T U V W X Y Z) do (
vol %%D: 2>nul | find "%targetSerial%" >nul
if !errorlevel! equ 0 (
set "usbDrive=%%D:\"
goto gotcha
)
)
:: If no matching drive is found
echo Flash drive with serial number %targetSerial% not found.
pause
exit /b
:gotcha
:: Check if the source folder exists on the USB drive, in our case it is ""
if not exist "%usbDrive%4upload\" (
echo Source folder "4upload" not found on the flash drive.
pause
exit /b
)
:: Copy .jpg files to the destination folder
xcopy "%usbDrive%4upload\*.jpg" "%destFolder%" /s /i /y
xcopy "%usbDrive%4upload\*.dng" "%destFolder%" /s /i /y
xcopy "%usbDrive%4upload\*.orf" "%destFolder%" /s /i /y
:: Add more extensions that should be processed
if errorlevel 1 (
echo Unknown error occurred while copying files.
) else (
echo Files copied successfully.
)
pause
BONUS PART:
If you want to move files that are not older than 8 days, make use of the wonderful Robocopy command.
Robocopy "%%D:\4upload" "%destFolder%" *.JPG /E /MOV /MAXAGE:8
Just put this line instead of:
xcopy "%usbDrive%4upload\*.jpg" "%destFolder%" /s /i /y
You can easily modify the scripts according to your personal needs.
For example, add more folders or file types, remove the pause, or change text messages.
Be sure to check out following related topics:
- HOW TO DELETE FOLDERS CREATED BY ANDROID ON SD-CARDS AND EXTERNAL DRIVES WITH ONE CLICK OR KEYSTROKE
- USEFUL SIMPLE BATCH FILES WHICH I USE ON DAILY BASIS